WooCommerce Wholesale Prices Premium Getting Started Guide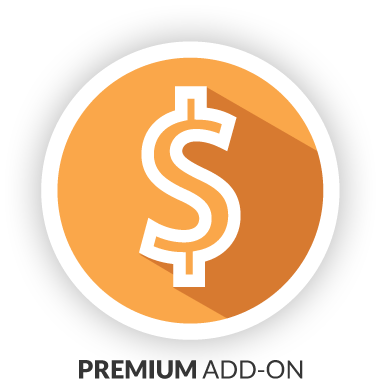
Thank you for purchasing the Premium add-on to our free Wholesale Prices plugin!
This document is a getting started guide for the WooCommerce Wholesale Prices Premium plugin for WooCommerce.
The Premium plugin lets you further reduce your reliance on clunky spreadsheets so all of your wholesale customers can enjoy ordering via your online store. It gives you a stack of extra features that will help you be more advanced about the way you offer wholesale products to your customers.
Here』s what this guide will cover:
Overview – we describe what the plugin does in addition to the free plugin
Additional Wholesale Customer Roles – we will show you how to add more user role levels so you can separate your wholesale customers into groups
More Wholesale Pricing Features – we show all about the extra flexible new ways you can apply wholesale pricing to your products
Product Visibility – show and hide products to both retail and wholesale customers and by their role as well
Shipping Mapping – this important feature will let you set up wholesale specific shipping
Payment Gateway Mapping – learn how to give your wholesale customers access to specific payment gateways
Minimum Order Requirements – set a minimum for activating wholesale pricing that you wholesale customers must meet
Advanced Tax – taxing is an important part of wholesale selling and we』ll show you how flexible you can make it
Other Plugins In The Suite – we』ll show you why you should consider adding Wholesale Order Form and Wholesale Lead Capture
Getting Help – we understand this can be an intricate tool, we』ll show you where to get help if you need it
Overview
WooCommerce Wholesale Prices Premium is the premium add-on plugin for the free WooCommerce Wholesale Prices plugin. It requires the free plugin to be installed and activated for it to work.
There are hundreds of features included in the premium add-on, you can see here for a full list, but today we』ll go over just a few of the major features so that you can get up and running as fast as possible.
Subscribe to Wholesale Suite
First, we』ll talk about the ability to add more wholesale roles and why you』d want to do that.
Additional Wholesale Roles
In the getting started guide for the free wholesale prices plugin we discussed why prices are attached to user roles.
In Prices Premium you can add additional user roles so you can do things like:
Create separate groups of wholesale customers
Define additional levels of pricing
Provide different minimum order requirements
And much more…
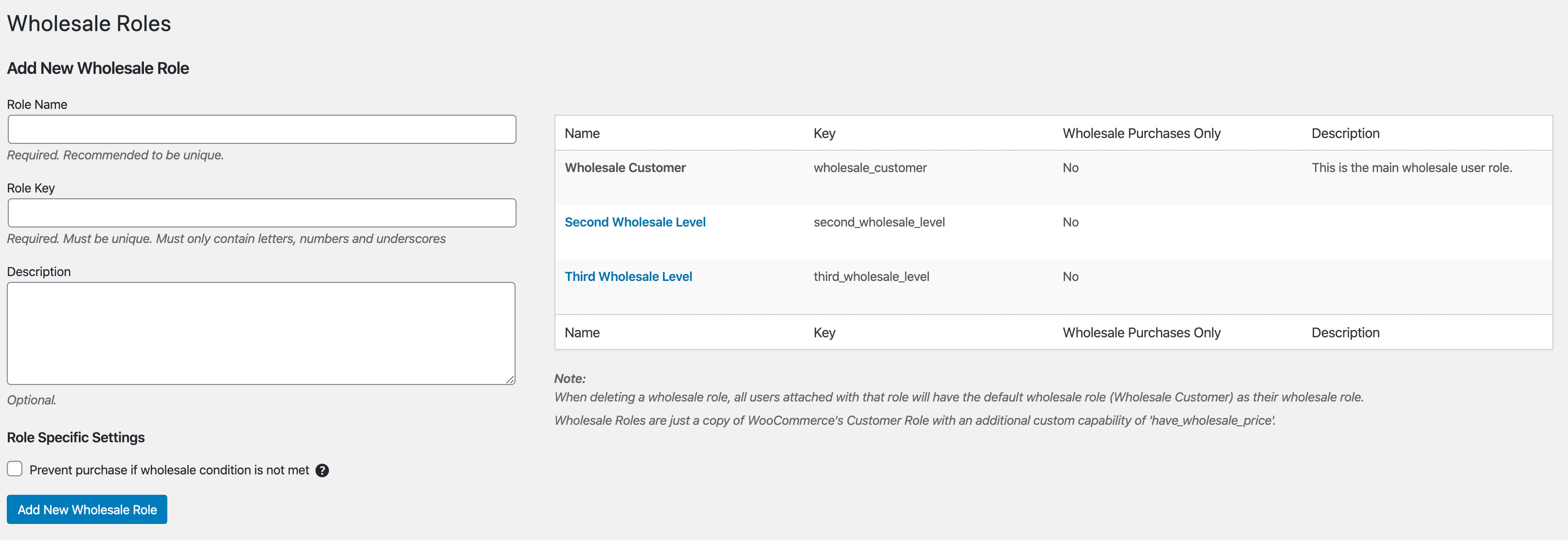
You can add extra roles easily via the WooCommerce->Wholesale Roles menu. It works a bit like a WordPress categories page so it should look familiar.
For each role added to the system, you will see wholesale pricing fields on your products.

There are two main reasons we』ve found that people add extra roles for:
They want to separate local wholesale customers from those that are from other countries so they can do different pricing, minimum levels, shipping methods, etc for those customers.
They want to create distinct 「levels」 of wholesale relationships, like Bronze, Silver, Gold, Platinum where the benefits and pricing get better as they go up the levels.
I encourage you to think through the structure you want for your wholesale customers before moving on to setting pricing.
Read this article for more in-depth instructions on how to add additional wholesale roles.
Percentage (%) Based Pricing & Quantity Based Pricing
In the free plugin, you can set wholesale pricing via the Product edit screen and for a lot of cases and this is fine for many stores.
But in the Prices Premium plugin, you can now also set wholesale pricing on the category level and the global level. You can also create quantity based pricing where the customer gets discounts on the price the more they buy.
We have a very detailed guide on how wholesale pricing works in Wholesale Suite here. Rather than re-iterate that detailed guide here, I will encourage you to go over to that article and get up to speed.
I will just explain one concept quickly though because it』s important. Wholesale prices follow rules of precedence which is roughly this:
Product Wholesale Price -> Category Level % Discount -> Global % Discount
If the Product』s wholesale price has not been set, the system will look to see if there is a category level % discount set, if not it will look to see if there is a global % discount. It always starts at the lowest level and stops when it finds that there has been a price defined.
So if you have defined both a global % discount that will be used across all of our products globally, but if you have set a specific price on a certain product that price will be used for that product.
Again, read the wholesale pricing guide and it will explain both this concept and go into details about how to set those % based prices properly.
Product Visibility
In Prices Premium you can show and hide products from customers in a few exciting ways. It gives you lots of flexibility such as being able to make wholesale-only products that aren』t shown to retail customers or likewise being able to hide non-wholesale products from wholesale customers.
The first is a setting called 「Only Show Wholesale Products To Wholesale」 which basically does what it says. Once enabled, your wholesale customers will only be able to see a product if it has wholesale pricing defined (and yes, this includes % based pricing).
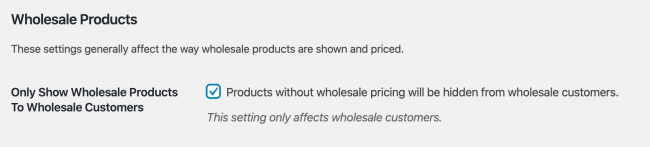
Next is a Product based setting which you will find on the Publish box of products called 「Restrict To Wholesale Roles」. Here you can restrict the visibility of a whole product to specific wholesale levels. This can be handy if you only open up certain products to certain customers, such as when you have separated customers by role geographically.
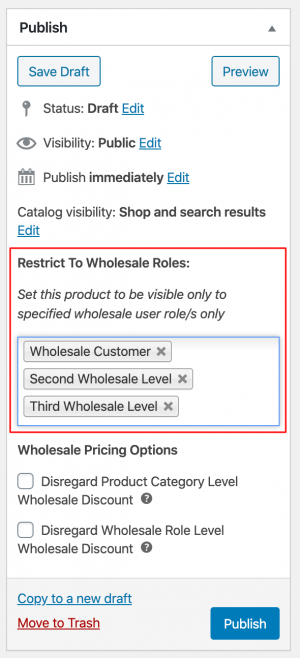
Lastly, you can also restrict the visibility of individual product variation on a variable type products. This can be done per role as well, much like the restrict to wholesale roles feature.

Again, we have an in-depth guide about product visibility here which you can dig into.
Shipping & Payment Gateway Mapping
One of the cool things about having users separated into user roles is that you can 「map」 things to those roles.
We do this in quite a few places, two major ones being the shipping mapping and the payment gateway mapping.
Shipping Method Mapping
Mapping a shipping method means that you can set the system to only show people on that wholesale role certain shipping methods. You can also hide those shipping methods so that they』re only visible to that particular wholesale role.
WooCommerce itself has excellent location-driven shipping controls. So you can have a shipping method for only a specific area.
Those two things combined mean that you can create specific wholesale shipping pricing per area per role.
A good example of this in use might be to create a 「Local Pickup」 shipping method for your local area only and also only make that available to wholesale customers.
Don』t worry, we have a full guide on how this shipping mapping works here. Once you get the hang of it, it』s not too complicated, but it can take a little while to set up if you have a lot of regions. Stick with it, it』s worth the extra time as in the long run you will be able to give wholesale customers accurate wholesale shipping rates.
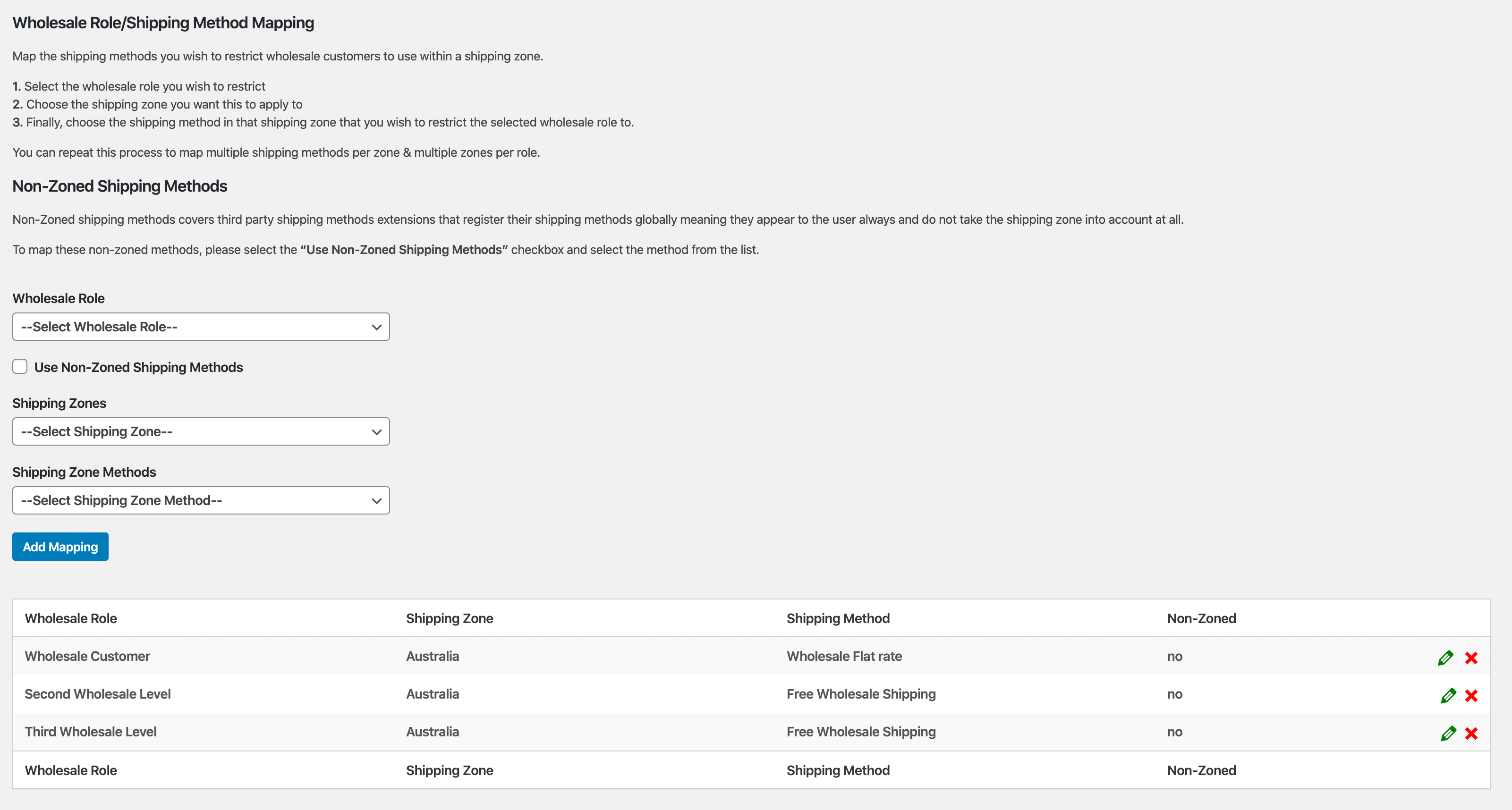
Payment Gateway Mapping
Not quite as complex as shipping method mapping, you can also apply the same mapping concept to payment gateways.
One thing to note is that you can map a disabled payment gateway. WooCommerce itself ensures that a disabled payment gateway will not show for customers. However, if you map it to a wholesale role, you can choose to have that payment gateway enable just for that wholesale role.
You can also map payment gateways to multiple wholesale roles.
A good example of this in use is you might want to enable the bank transfer payment gateway for wholesale customer roles, but not for retail customers.

Minimum Order Requirements
A very popular feature in Prices Premium is the ability to set minimum order requirements so that wholesale pricing doesn』t get activated unless your wholesale customer first satisfies a minimum amount in their cart.
Here』s what the settings look like:
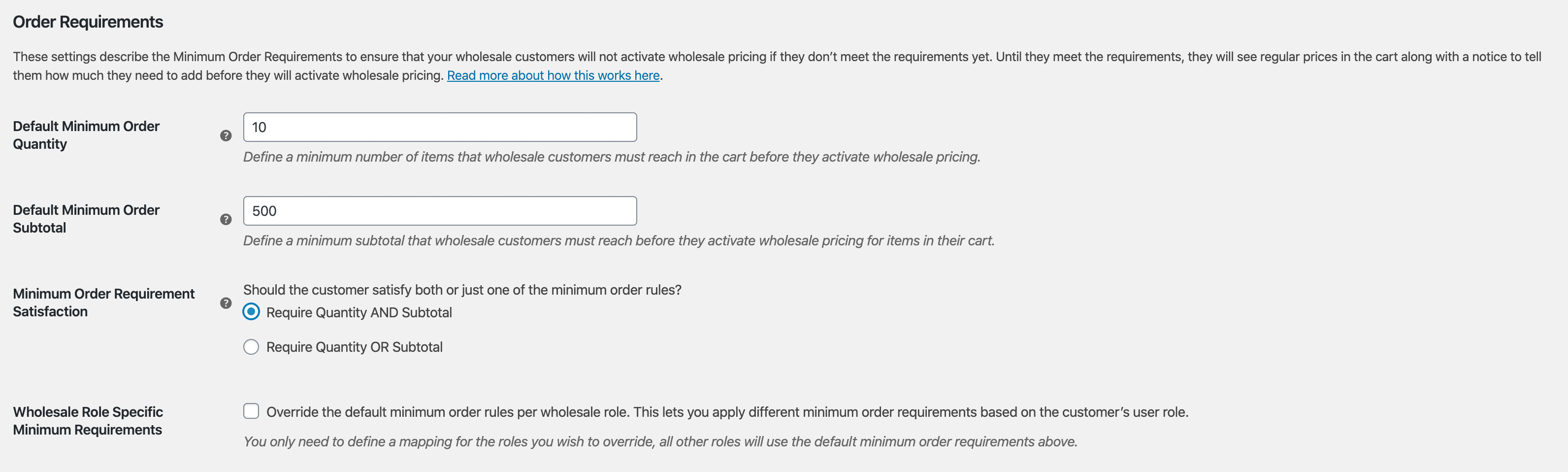
And here』s what the message looks like on the front end when they haven』t met their requirements yet:

You can also use the 「mapping」 concept here too so that you can override the minimum order restriction rules per wholesale role.
One extra note, it can be helpful to know that although customers can still add products to the cart the cart won』t reflect the wholesale price for those products until they meet the minimum.
This is because in many cases store owners still wish for wholesale customers to be able to buy a lower quantity if they really need to but they shouldn』t get wholesale pricing for those small orders. We go into depth about why this decision was made here.
If you wish you can also turn off the checkout button until they meet the restrictions if you wish.
Advanced Tax
Finally, we reach every world government』s favorite topic, taxation!
Understanding your business tax responsibilities is important when you enter into selling to wholesale customers. Some countries have specific rules that much be followed when it comes to the display of prices and their tax components.
WooCommerce handles tax based on location and for the most part, it does so fairly well. When it comes to tax, Prices Premium doesn』t replicate anything that WooCommerce does, it merely builds on it.
We effectively mirror the tax display options in WooCommerce itself, only for wholesale level customers:
Display wholesale prices in the store including or excluding tax (similar to the same for retail products)
Show a wholesale price suffix (similar to retail price suffix)
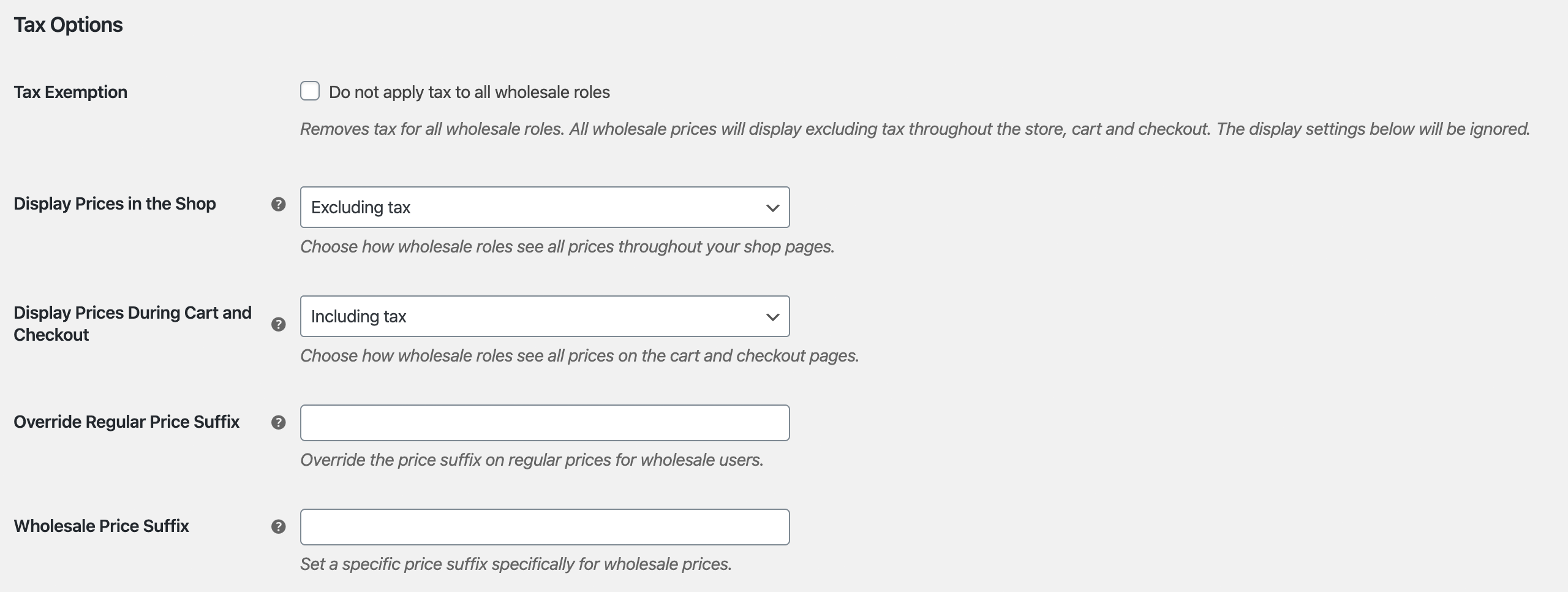
Another big thing we』ve added is the ability to do tax exemptions.
You can choose to do this globally, meaning all wholesale customers are tax exempt. Or you can, as you might have already guessed, map the wholesale exemptions per wholesale role.
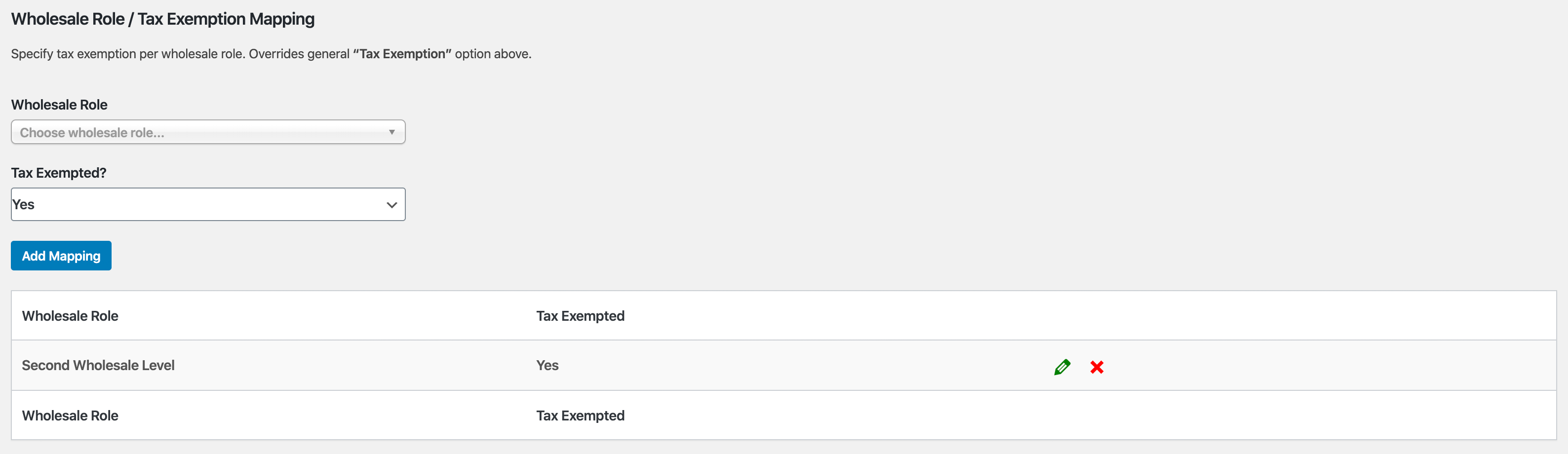
Advanced Features
When it comes to solving wholesale for WooCommerce we believe in a holistic approach. You need to solve all three of the big problems:
Setting wholesale prices, visibility, tax, etc. This is solved with Prices Premium as you』ve seen in this guide.
Efficient ordering – this is very important and greatly affects the happiness of your customers when they do business with you
Recruiting and managing wholesale level customers
WooCommerce Wholesale Order Form
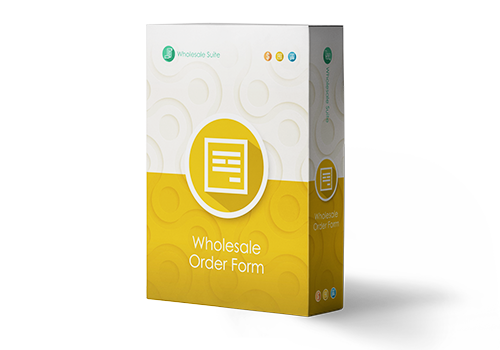
The time it takes for a wholesale customer to place an order is one of the biggest pain points you can eliminate.
Wholesale customers are not like regular retail customers. They don』t want to sift through your normal shop pages adding products to cart that way. They need a tabular form-based interface that makes it fast to add products to the cart in the quantities they desire.
This is where the Order Form plugin comes in.
Top Feature Highlights:
Your whole catalog on one page – searchable and categorized
No page reloads, full ajax enabled so wholesalers can add to cart without leaving the page
Slimline tabulated interface that is mobile & tablet friendly
Wildcard keyword & SKU searching
Hierarchical category filter
Permissions control so only those allowed can access the form
These are just a few high-level features that you』ll give your wholesale customers by using the Order Form plugin.
WooCommerce Wholesale Lead Capture
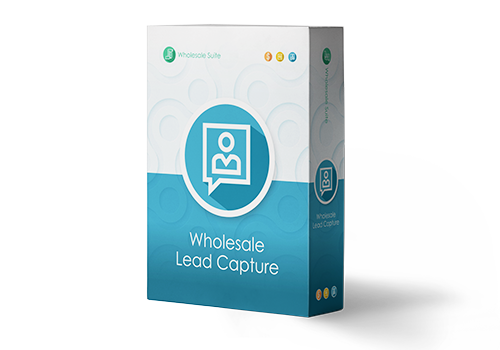
Managing wholesale level customers can be a bit of a headache if you are doing it manually. The Wholesale Lead Capture plugin gives you a wholesale specific registration form, dedicated wholesale login page, sign up email sequences, and your choice of manual or automated approvals.
It really takes the pain out of manually recruiting & registering wholesale customers which will save you time and let you put wholesale recruitment on autopilot.
Top Feature Highlights:
Registration form builder lets you capture whatever information you need during sign-up
Pre-fill the checkout fields with the information that you capture during sign up to smooth the first-order process for your new wholesale customer
Built-in spam protection with honeypot technology and optionally Google Recaptcha
Automated email sequences for admin and customer-facing approval and notification emails
Complete user approvals system which can be based on manual approvals or 100% automated if you wish
The Lead Capture plugin will make recruiting your wholesale customers and managing them much less painful.
Help & Support
We have a dedicated support team for Wholesale Suite who knows our products, WooCommerce, and the industry very well. You』re welcome to make use of their expertise at any time, worldwide.
If you are an existing customer please go to the support ticket request form and send us a message.
If you are a free plugin user, please send us a support request on the forum, we actively monitor the WordPress.org support forums for the free plugin and help our users there as best as we can.